Even more C#
1. Addresses and pointers#
In Java, you can manipulate the value of a variable via the program but not directly in memory (inside the JVM).
In C, you can retrieve the address of the location in memory where the variable is stored.
The operator
&
(reference of) represents the memory address of a variable.
Hands-on: Pointer
Inside the terminal, make suse that you are still inside
intro-c
, then usenano
to createpointer-1.c
with the source code below.
%p
is an output conversion syntax (similar to Java specifiers) for displaying memory address in hex format. See Other Output Conversions for more details.Compile and run
pointer-1.c
ls
gcc -o pointer-1 pointer-1.c
./pointer-1
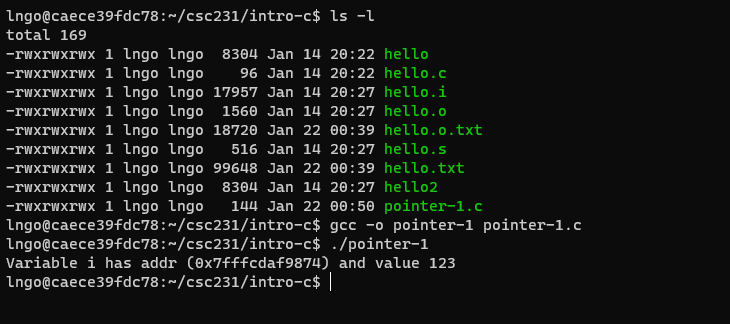
Pointer Definition
Pointer is a variable that points to a memory location (contains a memory location).
We call them pointer variables.
A pointer is denoted by a
*
character.The type of pointer must be the same as that of the value being stored in the memory location (that the pointer points to).
If a pointer points to a memory location, how do we get these locations?
An
&
character in front of a variable (includes pointer variables) denotes that variable’s address location.
Hands-on: Pointer and Variable’s Addresses
Inside the terminal, make suse that you are still inside
intro-c
, then usenano
to createpointer-2.c
with the source code below.
Since
p_i
is a pointer variable,p_i
contains a memory address (hence%p
).Then,
*p_i
will point to the value in the memory address contained in p_i.This is referred to as de-referencing.
This is also why the type of a pointer variable must match the type of data stored in the memory address the pointer variable contains.
Compile and run
pointer-2.c
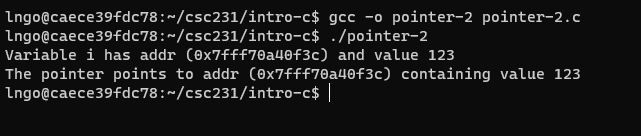
Pass by Value and Pass by Reference
Parameters are passed to functions.
Parameters can be value variables or pointer variables.
What is the difference?
Hands-on: Pass by value
Hands-on: Pass by reference
In Java, do you pass by value or pass by reference?
Answer
Primitives are passed by value.
Objects are passed by reference.
2. Pointers and memory allocation#
How does C request dynamic memory when you don’t know at compile-time exactly what you will need?
How does C allocate memory?
Automatic: compile arranges for memory to be allocated and initialized for local variables when it is in scope.
Static: memory for static variables are allocated once when program starts.
Dynamic: memory is allocated on the fly as needed.
Dynamic memory allocation
Unlike Java, you have to do everything!
Ask for memory.
Return memory when you are done (garbage collection!).
C function:
malloc
void *malloc(size_t size);
The
malloc()
function allocatessize
bytes and returns a pointer to the allocated memory. The memory is not initialized. If size is 0, thenmalloc()
returns eitherNULL
, or a unique pointer value that can later be successfully passed tofree()
.
C function:
free
void free(void *ptr);
The
free()
function frees the memory space pointed to by ptr, which must have been returned by a previous call tomalloc()
,calloc()
orrealloc()
. Otherwise, or iffree(ptr)
has already been called before, undefined behavior occurs. Ifptr
isNULL
, no operation is performed.
Void pointer
When
malloc
allocates memory, it returns a sequence of bytes, with no predefined types.A pointer that points to this sequence of bytes (the address of the starting byte), is called a void pointer.
A void pointer will then be typecast to an appropriate type.
Hands-on: malloc and type cast
Inside the terminal, make suse that you are still inside
intro-c
, then usenano
to createmalloc-1.c
with the source code below.
What points to where:
void *p = malloc(4);
: allocate 4 contiguous bytes. The address of the first byte is returned and assign to pointer variablep
.p
has no type, so it is avoid pointer
.int *ip = (int *)p;
: The address value pointed to byp
is assigned to pointer variableip
. The bytes pointed to bep
are now casted to typeint
.
Compile and run
malloc-1.c

Hands-on: malloc and type cast with calculation
Hands-on: Safety
Dynamic memory allocation
Critical to support complex data structures that grow as the program executes.
In Java, custom classes such as ArrayList and Vector provide such support.
In C, you have to do it manually: How?
Let’s start with a simpler problem:
How can we dynamically allocate memory to an array whose size is not known until during run time?
7. Array in C#
Inside the terminal, make suse that you are still inside
intro-c
, then usenano
to createarray-1.c
with the source code below.
What is the distance between addresses? Why?
Compile and run
array-1.c
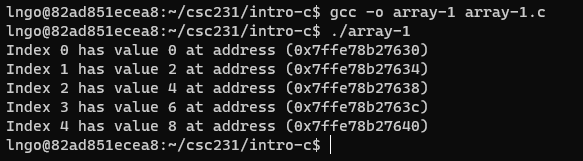
Exercise
An array variable
… is in fact pointing to an address containing a value.
… without the bracket notation and an index points to the corresponding address of the value at the index.
… is quite similar to a pointer!
8. Command line arguments.#
Inside the terminal, make suse that you are still inside
intro-c
, then usenano
to createarray-4.c
with the source code below.
In C, the command line arguments include the program’s name. The actual arguments start at index position 1 (not 0 like Java).
Compile and run
array-4.c
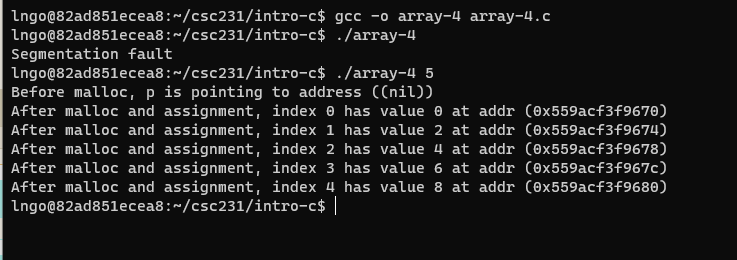
9. String#
Inside the terminal, make suse that you are still inside
intro-c
, then usenano
to createstring-1.c
with the source code below.
Compile and run
string-1.c

In C, string is considered an array of characters.
How many characters were printed out on the second line in the terminal?
Hint: Can you see all of them?
Answer
24
10. Object in C#
C has no classes or objects.
Instead, it has
struct
type (think ancestor of objects) .
Hands-on: Struct in C
Challenge
Modify
struct-1.c
so that it prints out the address oforigin
variable.What do you learn from the printed out addresses?
Answer
Insert printf("The address of the origin is: %p\n", &origin);
between the existing
printf
calls.
11. Function in C#
Almost the same as methods in Java, except for one small difference.
They need to either be declared, or must be defined prior to being called (relative to written code position).